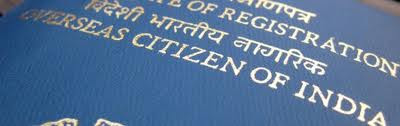
Citizenship Amendment Act, 2005
- The Citizenship Amendment Act, 2005 was passed by the Parliament to make provisions for dual citizenship by amending the Citizenship Act, 1955.
- The Citizenship Amendment Act, 2005, is to bestow eligibility for registration as Overseas Citizens of India (OCI) on persons of Indian origin, who or whose parents/grandparents have migrated from India after January 26, 1950 or were eligible to become an Indian Citizen on January 26, 1950 or belonged to a territory that became part of India after August 15, 1947, and their minor child, who is a national of a country that allows dual citizenship in some form or other.
- The eligibility provision is being extended to such citizens of all countries other than those who had ever been a citizen of Pakistan and Bangladesh.
Overseas Citizenship of India (OCI)
Eligibility for OCI
- Any person who at any time held an Indian Passport, or either he or one of his parents or grandparents was born in or was a permanent resident in India as defined in the Government of India Act, 1935 and other territories that became part of India thereafter provided he was at any time a citizen of Afghanistan, Bhutan, China, Nepal, Pakistan and Sri Lanka or who is a spouse of a citizen of India or a person of Indian
- A foreign national, who was eligible to become citizen of India on 26.01.1950 or was a citizen of India on or at anytime after 26.01.1950 or belonged to a territory that became part of India after 16.08.1947 and his/her children and grandchildren, provided his/her country of citizenship allows dual citizenship in some form or other under the local laws, is eligible for registration as Overseas Citizen of India (OCI).
1. Minor children of such a person are also eligible for OCI.
2. If the applicant had ever been a citizen of Pakistan or Bangladesh, he/she will not be eligible for OCI.
Procedure of acquiring OCI
- Eligible persons have to apply in the prescribed form along with enclosure form online.
- Applicant can apply to the Indian Mission/Post in the country where applicant is ordinarily residing.
- If applicant is in India on long term visa then to FRRO, Delhi, Mumbai, Kolkata, Amritsar, Chennai or to the Joint Secretary (Foreigners) MHA.
- Fee for getting OCI is Rs. 15,000 or equivalent in local currency for adults and for children upto 18 years is Rs. 7,500 or equivalent in local currency
Benefits to OCI holders
- A multi-entry, Multi-purpose life-long visa for visiting India.
- Exemption from the requirements of registration if they stay on any single visit in India which does not exceed 180 days.
- Parity with NRIs in respect of all facilities available to the latter in the economic, financial and educational fields except in matters relating to the acquisition of agricultural/plantation properties. No parity shall be allowed in the sphere of political rights.
- A person registered as OCI is eligible to apply for grant of Indian Citizenship under Section 5(l)(g) of the Citizenship Act, 1955. If he/she is registered as OCI for five years and has been residing in India for one year out of the five years making the application.
- OCI is not entitled to vote, be a member of Legislative Assembly or Legislative Council or Parliament and cannot hold constitutional posts such as President, Vice-President, Judge of the Supreme Court or High Courts and can not also normally hold employment in the Government.